How to Build Scalable Node.js Apps

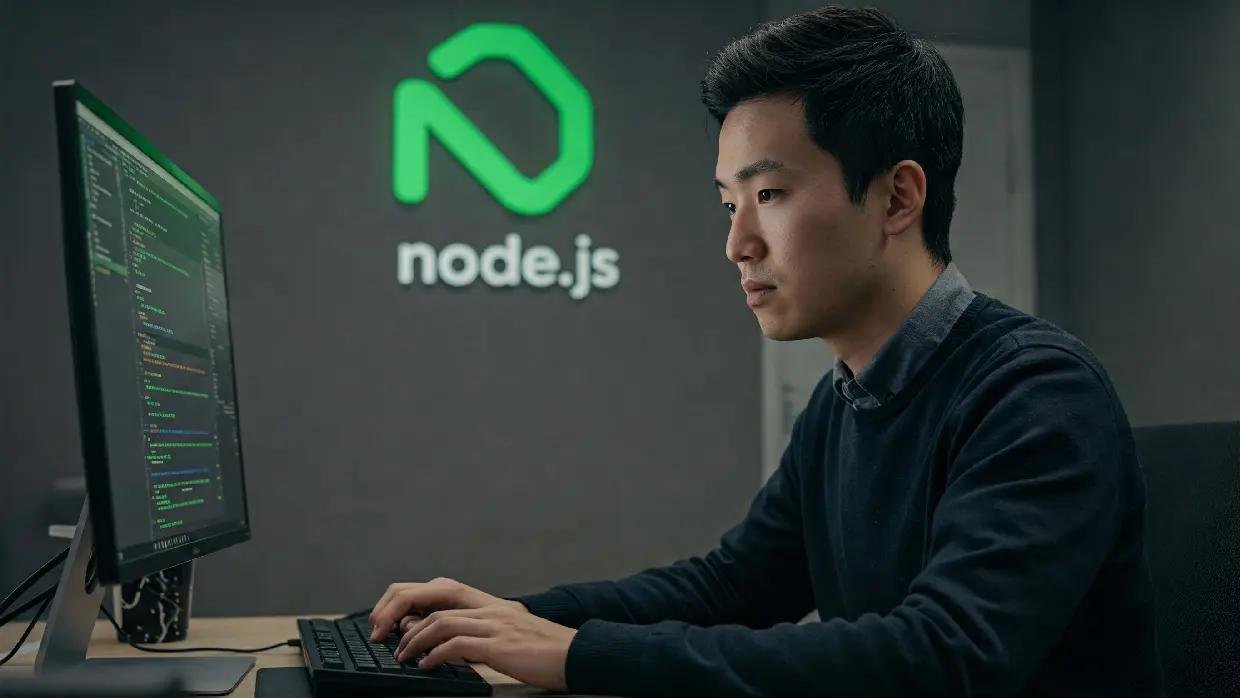

Scalable Node.js Applications: The Key to Handling Growing Traffic
Node.js, with its asynchronous, event-driven architecture, is an excellent choice for building applications that require high performance and scalability. However, to fully exploit the potential of Node.js, appropriate strategies and techniques must be applied. This article provides a comprehensive guide to building scalable Node.js applications.
1. Application Architecture
- Microservices: Instead of a monolithic application, consider a microservices-based architecture. Divide the application into smaller, independent services that can be developed, deployed, and scaled independently of each other.
- API Gateway: Use an API Gateway to manage request routing to different microservices and provide a unified interface for clients.
- Message Queue: Utilize message queues (e.g., RabbitMQ, Kafka) for asynchronous communication between microservices. This allows for decoupling tasks and improving reliability.
2. Clustering and Load Balancing
- Clustering: Node.js has a built-in
cluster
module that allows you to run multiple instances of the application on a single server. Each instance runs in a separate process, allowing you to utilize all processor cores and increase performance. - Load Balancing: Use a load balancer (e.g., Nginx, HAProxy) to evenly distribute traffic between different instances of the application. The load balancer monitors the status of instances and redirects traffic only to those that are available.
- PM2: PM2 is a process manager for Node.js that simplifies managing applications in a production environment. It automatically restarts applications after a crash, monitors their status, and allows for easy scaling.
3. Code Optimization
- Asynchronicity: Use asynchronous operations (e.g.,
async/await
, Promise) instead of synchronous ones to avoid blocking the event loop and ensure smooth application operation. - Avoiding Blocking Operations: Avoid performing operations that block the event loop, such as intensive calculations or long-term I/O operations. Move these operations to separate threads (e.g., using
worker_threads
). - Streaming: Utilize streaming to process large files or data streams. Streaming allows you to process data in batches instead of loading it entirely into memory.
- Caching: Implement caching at various levels (e.g., browser cache, server cache, database cache) to reduce server load and speed up data loading.
- Profiling: Use profiling tools (e.g., Node.js Inspector, Clinic.js) to identify bottlenecks in the code and optimize performance.
4. Databases
- Choosing the right database: Choose a database that best suits your needs. Consider NoSQL databases (e.g., MongoDB, Cassandra) for applications that require high scalability and flexibility.
- Connection Pooling: Use connection pooling to limit the number of connections to the database and optimize resource utilization.
- Indexing: Create appropriate indexes in the database to speed up data retrieval.
- Read Replicas: Use read replicas in the database to distribute the load between multiple servers and improve read performance.
5. Monitoring and Alerting
- Centralized Logging: Implement centralized logging (e.g., using ELK Stack) to easily monitor the status of the application and diagnose problems.
- Metrics Monitoring: Monitor key metrics (e.g., CPU usage, memory, response time) to quickly identify performance issues.
- Alerting: Configure an alerting system that will notify you of problems with the application (e.g., high load, errors).
- Health Checks: Implement health checks to monitor the status of application instances and automatically remove those that are unavailable.
6. Containerization and Orchestration
- Docker: Use Docker to containerize Node.js applications. Containers provide a consistent runtime environment and facilitate deployment.
- Kubernetes: Use Kubernetes to orchestrate Docker containers. Kubernetes automates the deployment, scaling, and management of containers.
- CI/CD: Implement a CI/CD (Continuous Integration/Continuous Deployment) process to automatically build, test, and deploy applications.
7. Security
- Regular Updates: Regularly update Node.js and dependencies to patch security vulnerabilities.
- OWASP Top 10: Familiarize yourself with the OWASP Top 10 list and secure the application against the most common attacks.
- Input Validation: Validate input data to prevent attacks such as SQL Injection and Cross-Site Scripting (XSS).
- Rate Limiting: Use rate limiting to limit the number of requests from one IP address and prevent DDoS attacks.
Sample Implementations
-
Using PM2 for Clustering:
pm2 start app.js -i max
-i max
runs as many instances of the application as there are processor cores. -
Nginx Configuration as a Load Balancer:
upstream backend { server app1:3000; server app2:3000; server app3:3000; } server { listen 80; location / { proxy_pass http://backend; } }
Summary
Building scalable applications with Node.js requires considering many factors, from architecture and code optimization to monitoring and security. By following the above practices, you can build applications that are ready for growing traffic and complexity, ensuring reliability and performance. Remember that scaling is a continuous process that requires regular monitoring and optimization.